iOS applications are pioneers in all categories, dealing with sensitive user data, payment data, and business data. They are the most susceptible applications to be targeted by cyber attacks disguised as data leaks, reverse engineering, and malware attacks. In the interest of offering secure protection for sensitive data, compliance with the guidelines, and trust on the part of the users, the security of an iOS application becomes a point of concern. This article puts iOS app security best practices in the spotlight, i.e., the most important aspects of secure coding practices, data security, network security, authentication features, and live monitoring.
iOS App Security Best Practices
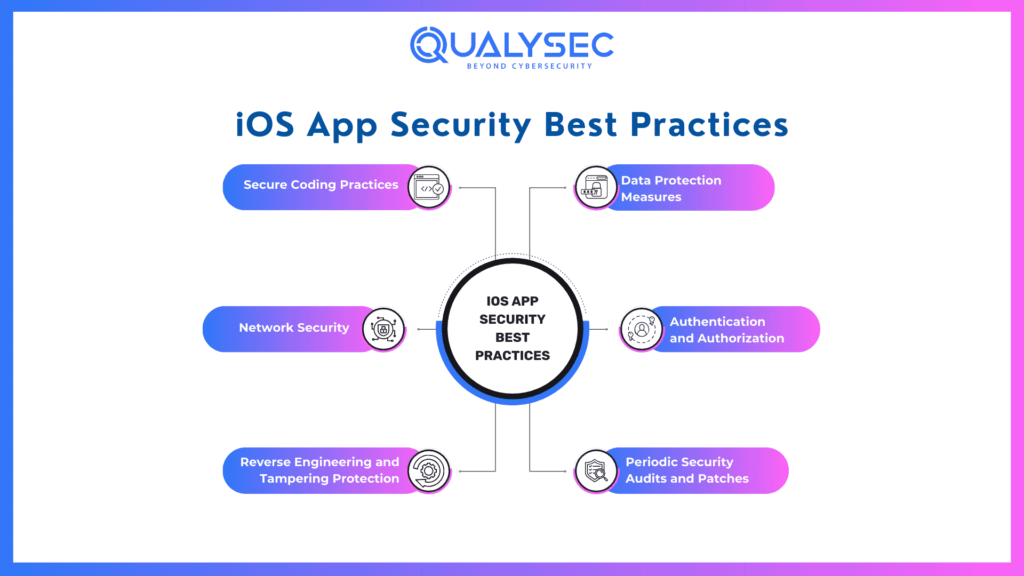
1. Secure Coding Practices
Secure coding is the backbone of iOS app security. The developers need to follow secure coding practices in such a manner that no one can take advantage of any weakness against them.
1.1 Do Not Hardcode Sensitive Data
Hardcoding sensitive values like API keys, auth tokens or credentials makes apps vulnerable to security attacks. These values can be obtained with ease by attackers through static analysis of app source code. Prevent this by:
- Saving sensitive values in secure storage containers like the iOS Keychain, which provides encryption and access control.
- Employing environment variables and server-side authentication for securing credentials.
1.2 Use Correct Data Types and Memory Management
Memory mismanagement bugs like buffer overflow and memory leaks can introduce security loopholes. To prevent such an attack:
- Use Automatic Reference Counting (ARC) of Swift with optimum efficiency for memory management.
- Do not use unsafe memory management at all, but utilize safe data types and robust input checking.
- Use secure programming techniques like bounds checking and sanitizing the input to dissuade exploitation.
1.3 Do Not Reverse Engineer
Reverse engineering enables the hackers to reverse-engineer application logic and gain access to sensitive data. To avoid it:
- Employ code obfuscation techniques such as **SwiftShield** to stop intruders from decompiling the source code.
- Remove debugging symbols from the release build using the `strip` tool to prevent internal structure leakage.
- Turn on bit code within Xcode so that Apple can recompile the application with patches and reduce the threat of exploits.
2. Data Protection Measures
iOS has several safeguards that are in place to guard user data. These are things that need to be done so that data doesn’t end up in the wrong hands and access.
2.1 Encrypt Secret Data
Encryption guards secret data from ending up in the wrong hands even when intercepted or accessed. The best practice is:
- Using AES-256 encryption to store sensitive data locally or in the database.
- Employing end-to-end encryption (E2EE) client-server for confidentiality of data.
2.2 Store Securely
Secure storage of sensitive information can itself be a security risk. For protection against threats:
- Store tokens, passwords, and other sensitive information in the iOS Keychain, which is secure and hardware-protected.
- Never store sensitive information in UserDefaults or local storage since they are not encrypted.
- Use the feature of File Protection API and apply protection level `NSFileProtectionComplete` to secure files, i.e., they are secured when the device is locked.
2.3 Data Leakage Prevention
Leakage is possible on non-standard media too. Misuse must be prevented:
- Disable keyboard caching for password input through `UITextInputTraits` to prevent sensitive input from being cached.
- Invoke `Pasteboard.protect()` to block sensitive data from being copied onto the pasteboard and pasted into a different app.
- Enable App Transport Security (ATS) to bake secure HTTPS connections, and data confidentiality and integrity will be maintained as it moves.
Implementation of these best practices facilitates iOS application developers to safeguard their applications, user information, and industry compliance. Implementation of storage best practices, encryption algorithms, and implementation of secure coding practices are best practices that prevent security vulnerabilities and ensure a secure user experience.
In-Depth iOS App Security Guide
Mobile apps these days handle huge amounts of sensitive user data, and security is a top priority. Threat actors are always on the lookout for app weaknesses to take advantage of and generate data breaches, identity theft, and financial fraud. As an iOS app developer, security best practices are of the highest order in an attempt to protect your users and also follow industry standards.
Securing an iOS app comprises different levels, from data encryption to safe means of authentication. This tutorial will guide you on the best practices of how to enhance security for your iOS app and protect it from dangerous threats.
“Read our comprehensive Guide to iOS Application Penetration Testing to gain valuable insights.
3. Network Security
Network security is required to protect against cyber attacks like Man-in-the-Middle (MITM) attacks, eavesdropping, and data interception. The attacker will attempt to intercept or manipulate data that is being sent from the app to a server. The application of suitable security features will ensure secure communication.
3.1 Apply HTTPS with App Transport Security (ATS)
- App Transport Security (ATS) is an Apple-implemented security feature that requires the use of Transport Layer Security (TLS) 1.2 or higher for all networking activity in iOS apps. Data is thus encrypted as it moves along the internet.
- ATS is on by default for iOS apps. It should never be disabled by developers except when they have no alternative.
- If exceptions are inevitable, don’t overgeneralize them. Restrict HTTP connections to only those domains that need it.
3.2 Implement SSL Pinning
- SSL Pinning defends against MITM attacks by causing the app to only talk to a trusted server. Rather than trusting certificate authorities installed on the device entirely, SSL pinning checks the server’s SSL certificate directly.
- Implement SSL pinning via libraries such as TrustKit.
Hash the server certificate and check against a stored value before accepting any connections.
Example SSL Pinning code:
let certificates = “YOUR_SERVER_PUBLIC_KEY_HASH”
func urlSession(_ session: URLSession, didReceive challenge: URLAuthenticationChallenge, completionHandler: @escaping (URLSession.AuthChallengeDisposition, URLCredential?) -> Void) {
if let serverTrust = challenge.protectionSpace.serverTrust, let certificate = SecTrustGetCertificateAtIndex(serverTrust, 0) {
let certData = SecCertificateCopyData(certificate) as Data
let certHash = sha256(data: certData)}
if certHash == certificateHash
completionHandler(.useCredential, URLCredential(trust: serverTrust))
return
}
}
completionHandler(.cancelAuthenticationChallenge, nil)
3.3 Use Secure APIs for Networking
- Utilize secure API calls to prevent unauthorized usage and data exposure.
- Use URLSession if they are security-audited third-party networking libraries.
- Implement encryption on all API requests and responses over HTTPS.
- Don’t hardcode API keys in the app code. Keep them securely in encrypted storage or fetch them from a server.
3.4 Protect WebViews
If your app makes use of WebViews, make sure they are set up correctly so that there are no exploits.
Disable JavaScript execution when not needed by:
WKPreferences().javaScriptEnabled = false
Limit URL loading so that the user can’t reach malicious websites. Block URLs with WKNavigationDelegate.
4. Authentication and Authorization
Authentication guarantees the presence of a user, whereas authorization guarantees that he is permitted to utilize the right resources. Flawed authentication systems result in unauthorized access, theft of data, and hijacked accounts.
4.1 Implement Multi-Factor Authentication (MFA)
Multi-factor authentication (MFA) adds a layer of protection where the user has to authenticate himself by using two or more factors, i.e.:
- One-Time Passwords (OTPs)
- Biometric login (Touch ID or Face ID)
- Hardware tokens
Implementation of Firebase Authentication or Auth0 makes implementing use of MFA a piece of cake to roll out in your app.
4.2 User Authentication Secure
- Password security against brute-force attacks and exposure of credentials.
- Hash passwords upon storing them with strong algorithms like Argon2, bcrypt, or PBKDF2.
- Never store passwords in plaintext within databases.
- Employ rate limiting to prevent sequential failed logins using the same IP address.
4.3 OAuth 2.0 Safe Use
- OAuth 2.0 is the most common method of secure authorization and authentication. Implement OAuth with:
- Don’t pass cached access tokens via URLs but rather Authorization headers.
- Use refresh tokens safely to periodically refresh user sessions without making them log in over and over again.
4.4 Use Session Expiry
- Implement session expiry to automatically log out idle users.
- Re-authenticate users from time to time, especially for high-risk activities such as financial transactions.
5. Reverse Engineering and Tampering Protection
Threat actors reverse-engineer software to discover security vulnerabilities, steal sensitive data, or alter software behavior for evil purposes. Software security can be significantly more difficult to reverse engineer if it is protected.
5.1 Turn Off Runtime Debugging
Threat actors use debugging features to monitor application runtime behavior. Disabling runtime debugging can prevent unauthorized runtime code alteration.
Example:
func isDebuggerAttached() -> Bool {
return getppid()!= 1
}
5.2 Identify Jailbroken Devices
Jailbroken devices can also be attacked with runtime attacks. Apps must be able to identify and restrict functionality on such devices when needed.
Example:
if FileManager.default.fileExists(atPath: “/Applications/Cydia.app”) {
print(“Device is jailbroken”)
}
5.3 Implement Runtime Protection
- Use iOS Hardened Runtime and Apple’s Notarization process to pin code against tampering.
- Implement third-party security SDKs like Arxan or Appdome as extra security.
6. Periodic Security Audits and Patches
Security is a continuous process. Audits and patches regularly ensure that vulnerabilities are discovered and fixed before they are exploited.
6.1 Periodic Penetration Testing
- Penetration testing will identify security loopholes before the attacker.
- Utilize scanners like Burp Suite, Frida, and MobSF for security scanning.
- Conduct ethical hacking tests to mimic real attack vectors.
6.2 Update Dependencies
- Legacy libraries and frameworks are riddled with security vulnerabilities.
- Update third-party dependencies regularly on a routine interval so fresh security patches are loaded.
- Test dependencies for security vulnerabilities with Snyk or OWASP Dependency-Check.
6.3 Log and Analyze App Activity
- Log the activity of the app so unusual activity can be traced.
- Utilize Apple’s Unified Logging System to perform high-volume logging.
- Implement Security Information and Event Management (SIEM) tools for real-time log viewing.
“Learn more with our detailed guide on the iOS Pentesting Checklist for step-by-step guidance.
Latest Penetration Testing Report
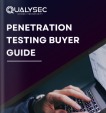
Conclusion
iOS app security is a practice of longevity with multi-layered security features like secure coding best practices, encryption, network protection, solid authentication, and live warnings. Developers are required to adhere to the best-in-class security methods by Apple, apps being updated timely, and security audits done so that the apps get hack-proofed.
By following these security practices, you can protect user data, guard against cyber attacks, and provide a secure and stable experience for your users.
iOS App Security Assistance Needed?
Want to make your iOS app more secure? We help with secure coding, penetration testing, and best security practice implementation. Let’s get your app secure, schedule a meeting today with our experts!
0 Comments